Members
ActionType
# actionType
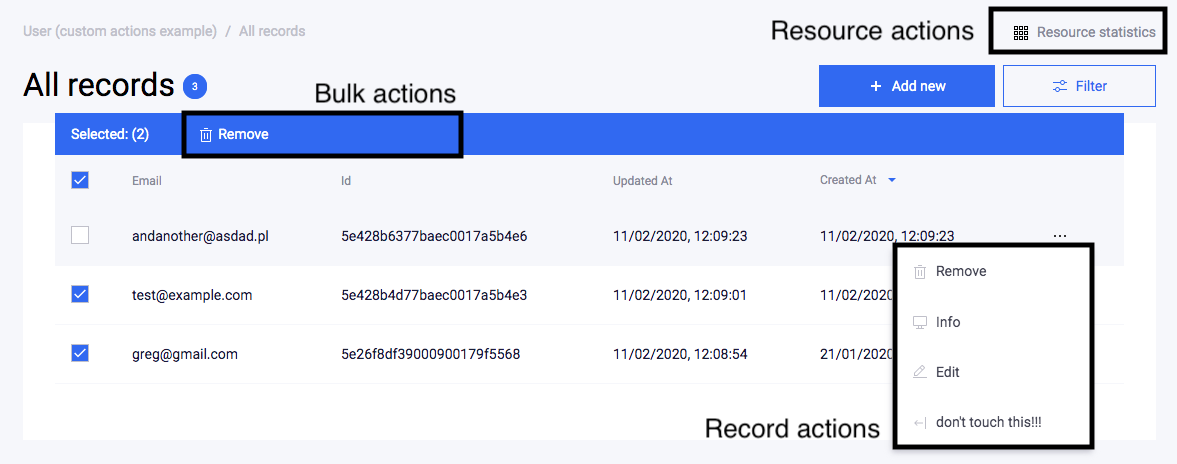
When you define a new action - it is required.
After.<T>
|
Array.<After.<T>>
# after Optional
by handler function response.
You can use it to (just an idea)
- create log of changes done in the app
- prefetch additional data after original Handler is being performed
Creating a changelog example:
// example mongoose model
const ChangeLog = mongoose.model('ChangeLog', mongoose.Schema({
// what action
action: { type: String },
// who
userId: { type: mongoose.Types.ObjectId, ref: 'User' },
// on which resource
resource: { type: String },
// was record involved (resource and recordId creates to polymorphic relation)
recordId: { type: mongoose.Types.ObjectId },
}, { timestamps: true }))
// actual after function
const createLog = async (originalResponse, request, context) => {
// checking if object doesn't have any errors or is a delete action
if ((request.method === 'post'
&& originalResponse.record
&& !Object.keys(originalResponse.record.errors).length)
|| context.action.name === 'delete') {
await ChangeLog.create({
action: context.action.name,
// assuming in the session we store _id of the current admin
userId: context.currentAdmin && context.currentAdmin._id,
resource: context.resource.id(),
recordId: context.record && context.record.id(),
})
}
return originalResponse
}
// and attaching this function to actions for all resources
const { ACTIONS } = require('adminjs')
ACTIONS.edit.after = [createLog]
ACTIONS.delete.after = [createLog]
ACTIONS.new.after = [createLog]
Before
|
Array.<Before>
# before Optional
method.
Example of hashing password before creating it:
actions: {
new: {
before: async (request) => {
if(request.payload.password) {
request.payload = {
...request.payload,
encryptedPassword: await bcrypt.hash(request.payload.password, 10),
password: undefined,
}
}
return request
},
}
}
string
|
false
# component Optional
use AdminJS.bundle method.
Action components accepts ActionProps and are rendered by the BaseActionComponent
When component is set to false
then action doesn't have it's own view.
Instead after clicking button it is immediately performed. Example of
an action without a view is module:DeleteAction.
string
|
number
|
Array.<(string|number)>
# containerWidth Optional
You can put here an actual size in px or an array of widths, where different values
will be responsible for different breakpoints.
It is directly passed to action's wrapping Box component, to its width
property.
Examples
// passing regular string
containerWidth: '800px'
// passing number for 100% width
containerWidth: 1
// passing values for different breakpoints
containerWidth: [1, 1/2, 1/3]
Record.<string, any>
# custom Optional
be stringified.
string
# guard Optional
new AdminJS({ resources: [{
resource: Car,
options: { actions: {
delete: {
guard: 'doYouReallyWantToDoThis',
}
}}
}]})
What you enter there goes to TranslateFunctions#translateMessage function, so in order to define the actual message you will have to specify its translation in AdminJSOptions.Locale
ActionHandler.<T>
|
Array.<ActionHandler.<T>>
|
null
# handler
- ApiController#resourceAction
- ApiController#recordAction
- or ApiController#bulkAction when user visits clicks action link.
If you are defining this action for a record it has to return:
- ActionResponse for resource action
- RecordActionResponse for record action
- BulkActionResponse for bulk action
// Handler of a 'record' action
handler: async (request, response, context) {
const user = context.record
const Cars = context._admin.findResource('Car')
const userCar = Car.findOne(context.record.param('carId'))
return {
record: user.toJSON(context.currentAdmin),
}
}
Required for new actions. For modifying already defined actions like new and edit we suggest using Action#before and Action#after hooks.
boolean
# hideActionHeader Optional
Action header consist of:
- breadcrumbs
- action buttons
- action title
string
# icon Optional
because what you put here is passed down to it.
new AdminJS({ resources: [{
resource: Car,
options: { actions: { edit: { icon: 'Add' } } },
}]})
boolean
|
IsFunction
# isAccessible Optional
You can pass a boolean or function of type IsFunction, which takes ActionContext as an argument.
You can use it as a carrier between the hooks.
Example for isVisible function which allows the user to edit cars which belongs only to her:
const canEditCars = ({ currentAdmin, record }) => {
return currentAdmin && (
currentAdmin.role === 'admin'
|| currentAdmin._id === record.param('ownerId')
)
}
new AdminJS({ resources: [{
resource: Car,
options: { actions: { edit: { isAccessible: canEditCars } } }
}]})
- See:
-
- ActionContext parameter passed to isAccessible
- IsFunction exact type of the function
View Source adminjs/src/backend/actions/action.interface.ts, line 241
boolean
|
IsFunction
# isVisible Optional
It also can be a simple boolean value.
True
by default.
The most common example of usage is to hide resources from the UI.
So let say we have 2 resources User and Cars:
const User = mongoose.model('User', mongoose.Schema({
email: String,
encryptedPassword: String,
}))
const Car = mongoose.model('Car', mongoose.Schema({
name: String,
ownerId: { type: mongoose.Types.ObjectId, ref: 'User' },
})
so if we want to hide Users collection, but allow people to pick user when creating cars. We can do this like this:
new AdminJS({ resources: [{
resource: User,
options: { actions: { list: { isVisible: false } } }
}]})
In contrast - when we use Action#isAccessible instead - user wont be able to pick car owner.
- See:
-
- ActionContext parameter passed to isAccessible
- IsFunction exact type of the function
View Source adminjs/src/backend/actions/action.interface.ts, line 205
LayoutElementFunction
|
Array.<LayoutElement>
# layout Optional
With the help of LayoutElement you can put all the properties to whatever layout you like, without knowing React.
This is an example of defining a layout
const layout = [{ width: 1 / 2 }, [
['@H3', { children: 'Company data' }],
'companyName',
'companySize',
]],
[
['@H3', { children: 'Contact Info' }],
[{ flexDirection: 'row', flex: true }, [
['email', { pr: 'default', flexGrow: 1 }],
['address', { flexGrow: 1 }],
]],
],
]
Alternatively you can pass a function taking CurrentAdmin as an argument. This will allow you to show/hide given property for restricted users.
To see entire documentation and more examples visit LayoutElement
BuildInActions
|
string
# name
If you use one of list, search, edit, new, show, delete or bulkDelete you override existing actions. For all other keys you create a new action.
string
# parent Optional
If parent action doesn't exists - it will be nested under name in the parent.
boolean
# showFilter Optional
Example of creating new resource action with filter
new AdminJS({ resources: [{
resource: Car,
options: { actions: {
newAction: {
type: 'resource',
showFilter: true,
}
}}
}]})
boolean
# showInDrawer Optional
boolean
# showResourceActions Optional
Defaults to true
VariantType
# variant Optional
Type Definitions
object
# ActionContext
Action#before and Action#after functions.
Apart from the properties defined below it also extends TranslateFunctions. So you can use i.e. context.translateMessage(...) and others...
Properties:
Name | Type | Attributes | Description |
---|---|---|---|
{...} |
TranslateFunction
|
all functions from TranslateFunctions interface. |
|
_admin |
AdminJS
|
current instance of AdminJS. You may use it to fetch other Resources by their names: |
|
resource |
BaseResource
|
Resource on which action has been invoked. Null for dashboard handler. |
|
record |
BaseRecord
|
<optional> |
Record on which action has been invoked (only for actionType === 'record') |
records |
Array.<BaseRecord>
|
<optional> |
Records on which action has been invoked (only for actionType === 'bulk') |
h |
ViewHelpers
|
view helpers |
|
action |
ActionDecorator
|
Object of currently invoked function. Not present for dashboard action |
|
currentAdmin |
CurrentAdmin
|
<optional> |
Currently logged in admin |
{...} |
any
|
Any custom property which you can add to context |
# async ActionHandler(request, response, context) → {Promise.<T>}
with ActionResponse, BulkActionResponse or RecordActionResponse
Parameters:
Name | Type | Description |
---|---|---|
request |
ActionRequest
|
|
response |
any
|
|
context |
ActionContext
|
Promise.<T>
object
# ActionRequest
Properties:
Name | Type | Attributes | Description |
---|---|---|---|
params |
object
|
parameters passed in an URL |
|
resourceId |
string
|
Id of current resource |
|
recordId |
string
|
<optional> |
Id of current record (in case of record action) |
recordIds |
string
|
<optional> |
Id of selected records (in case of bulk action) divided by commas |
action |
string
|
Name of an action |
|
query |
string
|
<optional> |
an optional search query string (for |
{...} |
any
|
||
payload |
Record.<string, any>
|
<optional> |
POST data passed to the backend |
query |
Record.<string, any>
|
<optional> |
Elements of query string |
method |
'post'
|
'get'
|
HTTP method |
object
# ActionResponse
Properties:
Name | Type | Attributes | Description |
---|---|---|---|
notice |
NoticeMessage
|
<optional> |
Notice message which should be presented to the end user after showing the action |
redirectUrl |
string
|
<optional> |
redirect path |
{...} |
any
|
Any other custom parameter |
# async After(response, request, context)
Parameters:
Name | Type | Description |
---|---|---|
response |
T
|
Response returned by the default ActionHandler |
request |
ActionRequest
|
Original request which has been sent to ActionHandler |
context |
ActionContext
|
Invocation context |
# async Before(request, context) → {Promise.<ActionRequest>}
method.
Parameters:
Name | Type | Description |
---|---|---|
request |
ActionRequest
|
Request object |
context |
ActionContext
|
Invocation context |
Promise.<ActionRequest>
object
# BulkActionResponse
Properties:
Name | Type | Description |
---|---|---|
records |
Array.<RecordJSON>
|
Array of RecordJSON objects. |
# IsFunction(context)
Parameters:
Name | Type | Description |
---|---|---|
context |
ActionContext
|
# LayoutElementFunction(currentAdminopt) → {Array.<LayoutElement>}
Parameters:
Name | Type | Attributes | Description |
---|---|---|---|
currentAdmin |
CurrentAdmin
|
<optional> |
Array.<LayoutElement>
object
# RecordActionResponse
Properties:
Name | Type | Description |
---|---|---|
record |
RecordJSON
|
Record object. |